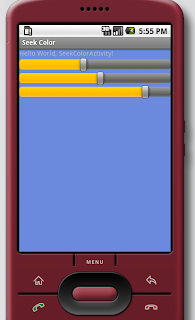
In this exercise, the background color is changed, controlled by SeekBar.
A SeekBar is an extension of ProgressBar that adds a draggable thumb. The user can touch the thumb and drag left or right to set the current progress level or use the arrow keys.
When SeekBarChange, the onProgressChanged() of OnSeekBarChangeListener will be called, and the background of the screen(LinearLayout) will be updated according to the value (SeekBar.getProgress()).
Create a Android Application, SeekColor, with three SeekBar for Red, Green and Blue setting.
main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:id="@+id/myScreen"
>
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/hello"
/>
<SeekBar
android:id="@+id/mySeekingBar_R"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:max="255"
android:progress="0"/>
<SeekBar
android:id="@+id/mySeekingBar_G"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:max="255"
android:progress="0"/>
<SeekBar
android:id="@+id/mySeekingBar_B"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:max="255"
android:progress="0"/>
</LinearLayout>
SeekColorActivity.java
package com.exercise.seekcolor;
import android.app.Activity;
import android.os.Bundle;
import android.widget.LinearLayout;
import android.widget.SeekBar;
public class SeekColorActivity extends Activity {
private int seekR, seekG, seekB;
SeekBar redSeekBar, greenSeekBar, blueSeekBar;
LinearLayout mScreen;
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
mScreen = (LinearLayout) findViewById(R.id.myScreen);
redSeekBar = (SeekBar) findViewById(R.id.mySeekingBar_R);
greenSeekBar = (SeekBar) findViewById(R.id.mySeekingBar_G);
blueSeekBar = (SeekBar) findViewById(R.id.mySeekingBar_B);
updateBackground();
redSeekBar.setOnSeekBarChangeListener(seekBarChangeListener);
greenSeekBar.setOnSeekBarChangeListener(seekBarChangeListener);
blueSeekBar.setOnSeekBarChangeListener(seekBarChangeListener);
}
private SeekBar.OnSeekBarChangeListener seekBarChangeListener
= new SeekBar.OnSeekBarChangeListener()
{
@Override
public void onProgressChanged(SeekBar seekBar, int progress,
boolean fromUser) {
// TODO Auto-generated method stub
updateBackground();
}
@Override
public void onStartTrackingTouch(SeekBar seekBar) {
// TODO Auto-generated method stub
}
@Override
public void onStopTrackingTouch(SeekBar seekBar) {
// TODO Auto-generated method stub
}
};
private void updateBackground()
{
seekR = redSeekBar.getProgress();
seekG = greenSeekBar.getProgress();
seekB = blueSeekBar.getProgress();
mScreen.setBackgroundColor(
0xff000000
+ seekR * 0x10000
+ seekG * 0x100
+ seekB
);
}
}

8 comments:
Thanks! This was really helpful in finding how to change the BG color mid-app.
hi,
how do you display minimum and maximum values for each seekbar?
hello Chaotic,
I'm not sure what you means!
Actually you know the min and max, you can make a TextView to display it.
best color control slider demo found yet. Thanks.
very nice demo
How if I use SeekBarPreference, and use key for set Background??
How to change seekbar color in acitivity???
You could also do:
mScreen.setBackgroundColor(Color.argb(255, seekR, seekG, seekB));
Post a Comment