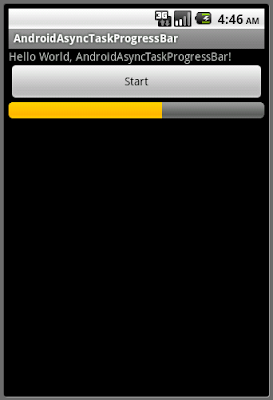
Modify main.xml to have a button to start the progress, and the ProgressBar.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
>
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/hello"
/>
<Button
android:id="@+id/startprogress"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Start"
/>
<ProgressBar
android:layout_width="fill_parent"
android:layout_height="wrap_content"
style="?android:attr/progressBarStyleHorizontal"
android:id="@+id/progressbar_Horizontal"
android:max="100"
/>
</LinearLayout>
Modify the java code.
package com.exercise.AndroidAsyncTaskProgressBar;
import android.app.Activity;
import android.os.AsyncTask;
import android.os.Bundle;
import android.os.SystemClock;
import android.view.View;
import android.widget.Button;
import android.widget.ProgressBar;
import android.widget.Toast;
public class AndroidAsyncTaskProgressBar extends Activity {
ProgressBar progressBar;
Button buttonStartProgress;
public class BackgroundAsyncTask extends
AsyncTask<Void, Integer, Void> {
int myProgress;
@Override
protected void onPostExecute(Void result) {
// TODO Auto-generated method stub
Toast.makeText(AndroidAsyncTaskProgressBar.this,
"onPostExecute", Toast.LENGTH_LONG).show();
buttonStartProgress.setClickable(true);
}
@Override
protected void onPreExecute() {
// TODO Auto-generated method stub
Toast.makeText(AndroidAsyncTaskProgressBar.this,
"onPreExecute", Toast.LENGTH_LONG).show();
myProgress = 0;
}
@Override
protected Void doInBackground(Void... params) {
// TODO Auto-generated method stub
while(myProgress<100){
myProgress++;
publishProgress(myProgress);
SystemClock.sleep(100);
}
return null;
}
@Override
protected void onProgressUpdate(Integer... values) {
// TODO Auto-generated method stub
progressBar.setProgress(values[0]);
}
}
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
buttonStartProgress = (Button)findViewById(R.id.startprogress);
progressBar = (ProgressBar)findViewById(R.id.progressbar_Horizontal);
progressBar.setProgress(0);
buttonStartProgress.setOnClickListener(new Button.OnClickListener(){
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
new BackgroundAsyncTask().execute();
buttonStartProgress.setClickable(false);
}});
}
}

Related articles:
- ProgressBar running in Runnable()
- AsyncTask: perform background operations and publish results on the UI thread
- onPostExecute() of AsyncTask
2 comments:
thanks for you article
this is my modification :)
thank for your article.
my modification :)
package com.example.asynctasktest;
import android.app.Activity;
import android.os.AsyncTask;
import android.os.Bundle;
import android.os.SystemClock;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.ProgressBar;
import android.widget.Toast;
public class MainActivity extends Activity {
//
private ProgressBar progress;
Button buttonStartProgress;
Button buttonCancel;
class ProgressTask extends AsyncTask {
@Override
protected void onPreExecute() {
// initialize the progress bar
//Toast, popup text when the progress is begin
Toast.makeText(MainActivity.this,
"Progress begin", Toast.LENGTH_LONG).show();
// set maximum progress to 100.
progress.setMax(100);
}
@Override
protected void onCancelled() {
// stop the progress back to 0
progress.setMax(0);
}
@Override
protected Void doInBackground(Integer... params) {
// get the initial starting value
int start = params[0];
// increment the progress
for (int i = start; i <= 100; i += 5) {
try {
boolean cancelled = isCancelled();
// if async task is not cancelled, update the progress
if (!cancelled) {
publishProgress(i);
// delay progress
SystemClock.sleep(1000);
}
} catch (Exception e) {
Log.e("Error", e.toString());
}
}
return null;
}
@Override
protected void onProgressUpdate(Integer... values) {
// increment progress bar by progress value
progress.setProgress(values[0]);
}
@Override
protected void onPostExecute(Void result) {
// async task finished
Log.v("Progress", "Finished");
//Toast, popup text when the progress is finshed
Toast.makeText(MainActivity.this,
"Progress finished", Toast.LENGTH_LONG).show();
//when the progress finished. the button can click again
buttonStartProgress.setClickable(true);
}
}
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
//call id function from main.xml
buttonStartProgress = (Button)findViewById(R.id.btn);
buttonCancel = (Button)findViewById(R.id.btnCancel);
progress = (ProgressBar)findViewById(R.id.progress);
//create click method function for start progress
buttonStartProgress.setOnClickListener(new Button.OnClickListener(){
@Override
public void onClick(View v) {
ProgressTask task=new ProgressTask();
switch(v.getId()){
case R.id.btn:
//execute progress starting from 10 to 100
task.execute(10);
//when the progressbarr still in progress. the button cant click
buttonStartProgress.setClickable(false);
break;
}
}
});
//create click method function for cancel progress
buttonCancel.setOnClickListener(new Button.OnClickListener(){
@Override
public void onClick(View v) {
ProgressTask task=new ProgressTask();
switch(v.getId()){
case R.id.btnCancel:
task.cancel(true);
break;
}
}
});
}
}
very well explained. Thank you.
Post a Comment