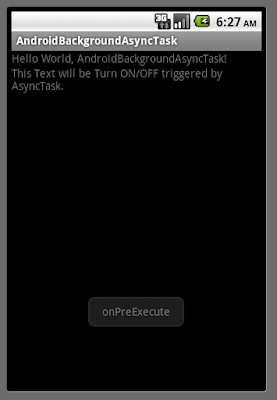
AsyncTask enables proper and easy use of the UI thread. This class allows to perform background operations and publish results on the UI thread without having to manipulate threads and/or handlers.
An asynchronous task is defined by a computation that runs on a background thread and whose result is published on the UI thread. An asynchronous task is defined by 3 generic types, called Params, Progress and Result, and 4 steps, called begin, doInBackground, processProgress and end.
main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
>
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/hello"
/>
<TextView
android:id="@+id/mytext"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="This Text will be Turn ON/OFF triggered by AsyncTask."
/>
</LinearLayout>
AndroidBackgroundAsyncTask.java
package com.exercise.AndroidBackgroundAsyncTask;
import android.app.Activity;
import android.os.AsyncTask;
import android.os.Bundle;
import android.os.SystemClock;
import android.view.View;
import android.widget.TextView;
import android.widget.Toast;
public class AndroidBackgroundAsyncTask extends Activity {
TextView myText;
public class BackgroundAsyncTask extends
AsyncTask<Void, Boolean, Void> {
boolean myTextOn;
@Override
protected Void doInBackground(Void... params) {
// TODO Auto-generated method stub
while(true)
{
myTextOn = !myTextOn;
publishProgress(myTextOn);
SystemClock.sleep(1000);
}
//return null;
}
@Override
protected void onPostExecute(Void result) {
// TODO Auto-generated method stub
//it will never been shown in this exercise...
Toast.makeText(AndroidBackgroundAsyncTask.this,
"onPostExecute", Toast.LENGTH_LONG).show();
}
@Override
protected void onPreExecute() {
// TODO Auto-generated method stub
myTextOn = true;
Toast.makeText(AndroidBackgroundAsyncTask.this,
"onPreExecute", Toast.LENGTH_LONG).show();
}
@Override
protected void onProgressUpdate(Boolean... values) {
// TODO Auto-generated method stub
if (values[0]){
myText.setVisibility(View.GONE);
}
else{
myText.setVisibility(View.VISIBLE);
}
}
}
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
myText = (TextView)findViewById(R.id.mytext);
new BackgroundAsyncTask().execute();
}
}

Related Article: onPostExecute() of AsyncTask
3 comments:
A quick question :
Could you explain the reason behind the comment " //it will never been shown in this exercise..." in onPostExecute()?
I am facing exactly the same problem, I do not get any exceptions, but my progress dialogs are never shown on the scree. I am wondering maybe its the same reason which made you write that comment.
Thanks!!
hello Mosh,
The method onPostExecute() will be called after return of doInBackground().
You can check my doInBackground() method, there is no return, it's a infinite loop. That's why the onPostExecute() method will not be called.
hello Mosh,
also refer onPostExecute() of AsyncTask.
Post a Comment