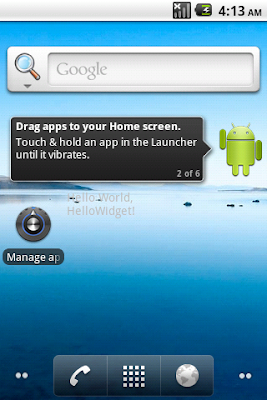
- Create a new project of ndroid application as normal, HelloWidget.
- Modify AndroidManifest.xml to have a receiver, with name of "HelloWidgetProvider", under Appliation.
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.exercise.HelloWidget"
android:versionCode="1"
android:versionName="1.0">
<application android:icon="@drawable/icon" android:label="@string/app_name">
<activity android:name=".HelloWidget"
android:label="@string/app_name">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<receiver android:name="HelloWidgetProvider" >
<intent-filter>
<action android:name="android.appwidget.action.APPWIDGET_UPDATE" />
</intent-filter>
<meta-data android:name="android.appwidget.provider"
android:resource="@xml/hellowidgetproviderinfo" />
</receiver>
</application>
<uses-sdk android:minSdkVersion="4" />
</manifest>
The <receiver> element requires the android:name attribute, which specifies the AppWidgetProvider used by the App Widget.The <intent-filter> element must include an <action> element with the android:name attribute. This attribute specifies that the AppWidgetProvider accepts the ACTION_APPWIDGET_UPDATE broadcast.
The <meta-data> element specifies the AppWidgetProviderInfo resource and requires the following attributes:
* android:name - Specifies the metadata name. Use android.appwidget.provider to identify the data as the AppWidgetProviderInfo descriptor.
* android:resource - Specifies the AppWidgetProviderInfo resource location.
- Create a new folder /res/xml, create a new hellowidgetproviderinfo.xml
<appwidget-provider xmlns:android="http://schemas.android.com/apk/res/android"
android:minWidth="146dp"
android:minHeight="72dp"
android:updatePeriodMillis="10000"
android:initialLayout="@layout/hellowidget_layout"
>
</appwidget-provider>
It define the AppWidgetProviderInfo object in an XML resource using a single <appwidget-provider>, include the essential qualities of an App Widget, such as its minimum layout dimensions, its initial layout resource, how often to update the App Widget, and (optionally) a configuration Activity to launch at create-time.- Create a layout file /res/layout/hellowidget_layout.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
>
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/hello"
/>
</LinearLayout>
It's the layout of the widget. In this dummy exercisse, just copy the content of the auto-generated layout, main.xml.- Create a class HelloWidgetProvider.java extends AppWidgetProvider. With nothing inside.
package com.exercise.HelloWidget;
import android.appwidget.AppWidgetProvider;
public class HelloWidgetProvider extends AppWidgetProvider {
}
It do nothing at all.Now you can build and Install the application as normal, then close it after started. It's not own target in this exercise.
- Add the HelloWidget on Home Screen, as describe in article "Home Screen App Widget". It's a dummy widget without any function, just show how to create a app widget.

Related Article:
minimum android:updatePeriodMillis
A simple Home Screen App Widget to get Date/Time
5 comments:
Hi, maybe you can help. I get the error: Class com.exercise.HelloWidget.HelloWidget does not exist.
And right, these class never gets defined, just HelloWidgetProvider.
What do I need to do to fix this? Just define an empty class HelloWidget?
Matthias
first try to delete the whole "activity" it should be working since its never defined.. or yes you can define an empty class HelloWidget.
ei can how can i insert a webview or an website in this widget?
thanks.
Juan
Hello Matthias,
May be because you have defined HelloWidget in AndroidManifest.xml without implemented the activity(HelloWidget.java).
You can:
- Just implemented a dummy HelloWidget.java.
or
- Delete the <activity> elements of HelloWidget in AndroidManifest.xml.
Hello travelbuff,
Refer to the "Creating the App Widget Layout" in "http://developer.android.com/guide/topics/appwidgets/index.html#CreatingLayout", App Widget can support following widget classes:
* AnalogClock
* Button
* Chronometer
* ImageButton
* ImageView
* ProgressBar
* TextView
In my understanding, WebView is not supported to be embedded in App Widget.
Post a Comment