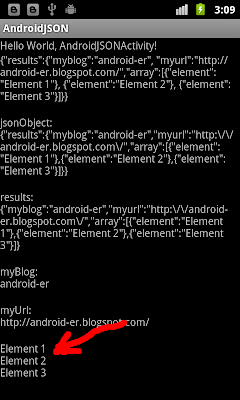
Modify res/values/strings.xml to add array in dummy JSON string.
<?xml version="1.0" encoding="utf-8"?>
<resources>
<string name="hello">Hello World, AndroidJSONActivity!</string>
<string name="app_name">AndroidJSON</string>
<string name="dummy_json">"{\"results\":{\"myblog\":\"android-er\", \"myurl\":"\""http://android-er.blogspot.com/\",\"array\":[{\"element\":\"Element 1\"}, {\"element\":\"Element 2\"}, {\"element\":\"Element 3\"}]"}}"</string>
</resources>
Modify main.xml to add a TextView "json_myarray" to display the array elements.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
>
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/hello"
/>
<TextView
android:id="@+id/json_src"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
<TextView
android:id="@+id/json_object"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
<TextView
android:id="@+id/json_result"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
<TextView
android:id="@+id/json_myblog"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
<TextView
android:id="@+id/json_myurl"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
<TextView
android:id="@+id/json_myarray"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
</LinearLayout>
Modify the code, to retrieve array elements from JSON.
package com.exercise.AndroidJSON;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import android.app.Activity;
import android.os.Bundle;
import android.widget.TextView;
import android.widget.Toast;
public class AndroidJSONActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
TextView JsonSrc = (TextView)findViewById(R.id.json_src);
TextView JsonObject = (TextView)findViewById(R.id.json_object);
TextView JsonResult = (TextView)findViewById(R.id.json_result);
TextView JsonMyBlog = (TextView)findViewById(R.id.json_myblog);
TextView JsonMyUrl = (TextView)findViewById(R.id.json_myurl);
TextView JsonMyArray = (TextView)findViewById(R.id.json_myarray);
String json_source = getString(R.string.dummy_json);
JsonSrc.setText(json_source);
try {
JSONObject jsonObject = new JSONObject(json_source);
JSONObject results = jsonObject.getJSONObject("results");
String myBlog = results.getString("myblog");
String myUrl = results.getString("myurl");
JsonObject.setText("\njsonObject:\n" + jsonObject.toString());
JsonResult.setText("\nresults:\n" + results.toString());
JsonMyBlog.setText("\nmyBlog:\n" + myBlog);
JsonMyUrl.setText("\nmyUrl:\n" + myUrl);
//Retrieve Array Elements in JSON
String stringArrayElement = "\n";
JSONArray jsonArray = results.getJSONArray("array");
for (int i = 0; i < jsonArray.length(); i++) {
JSONObject arrayElement = jsonArray.getJSONObject(i);
stringArrayElement += arrayElement.getString("element") + "\n";
}
JsonMyArray.setText(stringArrayElement);
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
Toast.makeText(AndroidJSONActivity.this,
e.toString(),
Toast.LENGTH_LONG).show();
}
}
}

Related article:
- Another example to parse JSON
1 comment:
wow... googd article man... very much
Post a Comment