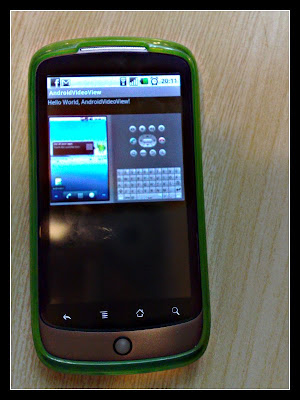
main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
>
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/hello"
/>
<VideoView
android:id="@+id/videoview"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
/>
</LinearLayout>
AndroidVideoView.java
package com.exercise.AndroidVideoView;
import android.app.Activity;
import android.net.Uri;
import android.os.Bundle;
import android.widget.MediaController;
import android.widget.VideoView;
public class AndroidVideoView extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
VideoView myVideoView = (VideoView)findViewById(R.id.videoview);
String viewSource ="rtsp://v5.cache1.c.youtube.com/CjYLENy73wIaLQklThqIVp_AsxMYESARFEIJbXYtZ29vZ2xlSARSBWluZGV4YIvJo6nmx9DvSww=/0/0/0/video.3gp";
myVideoView.setVideoURI(Uri.parse(viewSource));
myVideoView.setMediaController(new MediaController(this));
myVideoView.requestFocus();
myVideoView.start();
}
}
The App can run on real phone (tested on Nexus One), but cannot run on emulator with error of "Sorry, this video cannot be played", may be limited by the actual performance of emulator.
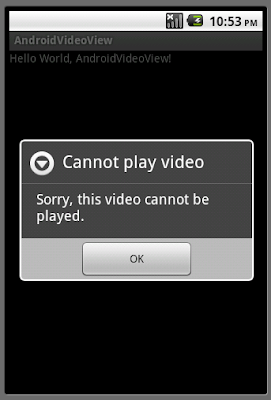
To capture the URL of YouTube video in 3gp, browse to http://m.youtube.com/ and capture the URL.

Related article:
- Event handler of VideoView - OnCompletion, OnPrepared and OnError
2 comments:
How to auto close player when video ended?
hello Dale,
You can implement your OnCompletionListener to detect video end. Please refer Event handler of VideoView - OnCompletion, OnPrepared and OnError.
Post a Comment