We can check if any package installed to handle the intent in advance by calling queryIntentActivities(). If the returned list not ">0" (no aplications installed), open Market to install "market://details?id=com.google.android.gm".
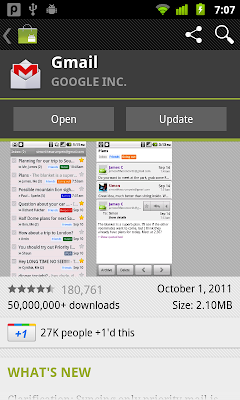
Such a approach can be applied to other intent.
package com.exercise.AndroidEMail;
import java.util.List;
import android.app.Activity;
import android.content.Intent;
import android.content.pm.PackageManager;
import android.content.pm.ResolveInfo;
import android.net.Uri;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
public class AndroidEMail extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
final EditText edittextEmailAddress = (EditText)findViewById(R.id.email_address);
final EditText edittextEmailSubject = (EditText)findViewById(R.id.email_subject);
final EditText edittextEmailText = (EditText)findViewById(R.id.email_text);
Button buttonSendEmail_intent = (Button)findViewById(R.id.sendemail_intent);
//Preset default
edittextEmailAddress.setText("---@gmail.com");
edittextEmailSubject.setText("test");
edittextEmailText.setText("email body");
buttonSendEmail_intent.setOnClickListener(new Button.OnClickListener(){
@Override
public void onClick(View arg0) {
// TODO Auto-generated method stub
String emailAddress = edittextEmailAddress.getText().toString();
String emailSubject = edittextEmailSubject.getText().toString();
String emailText = edittextEmailText.getText().toString();
String emailAddressList[] = {emailAddress};
Intent intent = new Intent(Intent.ACTION_SEND);
intent.setType("plain/text");
intent.putExtra(Intent.EXTRA_EMAIL, emailAddressList);
intent.putExtra(Intent.EXTRA_SUBJECT, emailSubject);
intent.putExtra(Intent.EXTRA_TEXT, emailText);
//Check if Intent available
List<ResolveInfo> list = getPackageManager().queryIntentActivities(intent, PackageManager.MATCH_DEFAULT_ONLY);
if (list.size() >0 ){
startActivity(Intent.createChooser(intent, "Choice App to send email:"));
}else{
//Start Market to downoad and install gmail
Uri uri = Uri.parse("market://details?id=com.google.android.gm");
Intent it = new Intent(Intent.ACTION_VIEW, uri);
startActivity(it);
}
}});
}
}
The layout, main.xml, refer to the article "Send email using Intent.ACTION_SEND".

No comments:
Post a Comment