Last exercise demonstrate how to "
Using GestureDetector with SimpleOnGestureListener". In this exercise, we modify the SimpleOnGestureListener to detect swipe by overriding onFling() method.
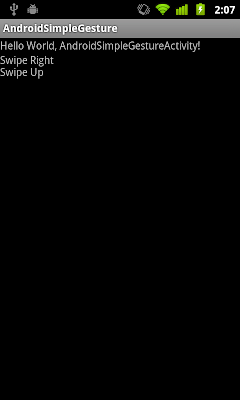
package com.exercise.AndroidSimpleGesture;
import android.app.Activity;
import android.os.Bundle;
import android.view.GestureDetector;
import android.view.GestureDetector.SimpleOnGestureListener;
import android.view.MotionEvent;
import android.widget.TextView;
public class AndroidSimpleGestureActivity extends Activity {
TextView gestureEvent;
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
gestureEvent = (TextView)findViewById(R.id.GestureEvent);
}
@Override
public boolean onTouchEvent(MotionEvent event) {
// TODO Auto-generated method stub
return gestureDetector.onTouchEvent(event);
}
SimpleOnGestureListener simpleOnGestureListener
= new SimpleOnGestureListener(){
@Override
public boolean onFling(MotionEvent e1, MotionEvent e2, float velocityX,
float velocityY) {
String swipe = "";
float sensitvity = 50;
// TODO Auto-generated method stub
if((e1.getX() - e2.getX()) > sensitvity){
swipe += "Swipe Left\n";
}else if((e2.getX() - e1.getX()) > sensitvity){
swipe += "Swipe Right\n";
}else{
swipe += "\n";
}
if((e1.getY() - e2.getY()) > sensitvity){
swipe += "Swipe Up\n";
}else if((e2.getY() - e1.getY()) > sensitvity){
swipe += "Swipe Down\n";
}else{
swipe += "\n";
}
gestureEvent.setText(swipe);
return super.onFling(e1, e2, velocityX, velocityY);
}
};
GestureDetector gestureDetector
= new GestureDetector(simpleOnGestureListener);
}
Download the files.
3 comments:
Dude. Your implementation worked for me.
This was really helpful for me. I spent a week looking for a way to successfully use swiping for my code. This worked beautifully.
got an error::::
main cannot be resolved or is not a field
Post a Comment