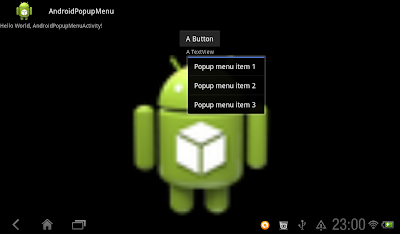
Create a file /res/menu/popupmenu.xml to define the PopupMenu.
<menu xmlns:android="http://schemas.android.com/apk/res/android">
<group android:id="@+id/group_popupmenu">
<item android:id="@+id/menu1"
android:title="Popup menu item 1"/>
<item android:id="@+id/menu2"
android:title="Popup menu item 2"/>
<item android:id="@+id/menu3"
android:title="Popup menu item 3"/>
</group>
</menu>
Main Java code
package com.AndroidPopupMenu;
import android.app.Activity;
import android.os.Bundle;
import android.view.MenuItem;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.ImageView;
import android.widget.PopupMenu;
import android.widget.TextView;
import android.widget.Toast;
public class AndroidPopupMenuActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
Button button = (Button)findViewById(R.id.button);
TextView text = (TextView)findViewById(R.id.text);
ImageView image = (ImageView)findViewById(R.id.image);
button.setOnClickListener(viewClickListener);
text.setOnClickListener(viewClickListener);
image.setOnClickListener(viewClickListener);
}
OnClickListener viewClickListener
= new OnClickListener(){
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
showPopupMenu(v);
}
};
private void showPopupMenu(View v){
PopupMenu popupMenu = new PopupMenu(AndroidPopupMenuActivity.this, v);
popupMenu.getMenuInflater().inflate(R.menu.popupmenu, popupMenu.getMenu());
popupMenu.setOnMenuItemClickListener(new PopupMenu.OnMenuItemClickListener() {
@Override
public boolean onMenuItemClick(MenuItem item) {
Toast.makeText(AndroidPopupMenuActivity.this,
item.toString(),
Toast.LENGTH_LONG).show();
return true;
}
});
popupMenu.show();
}
}
Main layout, main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical"
android:gravity="center_horizontal">
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/hello" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="A Button"
/>
<TextView
android:id="@+id/text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="A TextView"
/>
<ImageView
android:id="@+id/image"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:src="@drawable/ic_launcher"
/>
</LinearLayout>
Related:
- PopupWindow
3 comments:
widget PopupMenu is not taking on lower version so you should taking higher version of android.
Thanks & Regards,
Urvish Patel
You can use the support library v7 which contains the popupMenu.
To add the support library v7, just follow instructions in this link : http://developer.android.com/tools/support-library/setup.html#libs-with-res
I love simple example like this, and in my case it really works well. Thank you very much!
Post a Comment