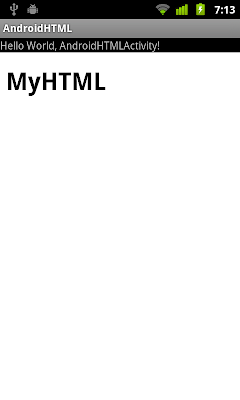
Create your own HTML file under /assets/ folder.
/assets/mypage.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width; user-scalable=0;" />
<title>My HTML</title>
</head>
<body>
<h1>MyHTML</h1>
</body>
</html>
Modify main.xml to add a WebView
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
>
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/hello"
/>
<WebView
android:id="@+id/mybrowser"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
/>
</LinearLayout>
Java code
package com.exercise.AndroidHTML;
import android.app.Activity;
import android.os.Bundle;
import android.webkit.WebView;
public class AndroidHTMLActivity extends Activity {
WebView myBrowser;
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
myBrowser = (WebView)findViewById(R.id.mybrowser);
myBrowser.loadUrl("file:///android_asset/mypage.html");
}
}

Related article:
- AndroidBrowser, implement a Android Browser using WebView
- Run Android Java code from Webpage
4 comments:
Thank you. It was useful:)
Nice!!!
To enable JavaScript use this way:
myBrowser = (WebView)findViewById(R.id.mybrowser);
myBrowser.getSettings().setJavaScriptEnabled(true);
myBrowser.loadUrl("file:///android_asset/mypage.html");
Nice!!!
To enable JavaScript use this way:
myBrowser = (WebView)findViewById(R.id.mybrowser);
myBrowser.getSettings().setJavaScriptEnabled(true);
myBrowser.loadUrl("file:///android_asset/mypage.html");
"myBrowser.getSettings().setJavaScriptEnabled(true);" is not working :( just black screen
Post a Comment