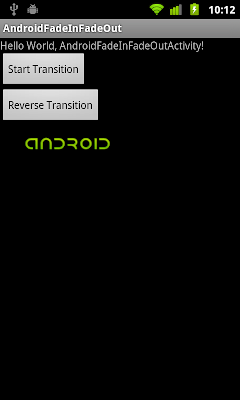
Modify main.xml to add a button to start reverse transition.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical" >
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/hello" />
<Button
android:id="@+id/starttransition"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Start Transition" />
<Button
android:id="@+id/reversetransition"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Reverse Transition" />
<ImageView
android:id="@+id/mytransition"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@anim/fadein" />
</LinearLayout>
Modify activity java code to call myTransitionDrawable.reverseTransition(1000) when button pressed.
package com.exercise.AndroidFadeInFadeOut;
import android.app.Activity;
import android.graphics.drawable.TransitionDrawable;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.ImageView;
public class AndroidFadeInFadeOutActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
ImageView myImage = (ImageView)findViewById(R.id.mytransition);
final TransitionDrawable myTransitionDrawable = (TransitionDrawable)myImage.getDrawable();
Button startTransition = (Button)findViewById(R.id.starttransition);
startTransition.setOnClickListener(new Button.OnClickListener(){
@Override
public void onClick(View arg0) {
// TODO Auto-generated method stub
myTransitionDrawable.startTransition(1000);
}});
Button reverseTransition = (Button)findViewById(R.id.reversetransition);
reverseTransition.setOnClickListener(new Button.OnClickListener(){
@Override
public void onClick(View arg0) {
// TODO Auto-generated method stub
myTransitionDrawable.reverseTransition(1000);
}});
}
}

No comments:
Post a Comment