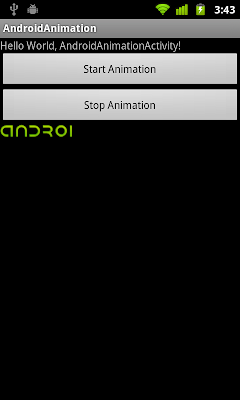
package com.exercise.AndroidAnimation;
import android.app.Activity;
import android.graphics.drawable.AnimationDrawable;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.ImageView;
public class AndroidAnimationActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
ImageView myAnimation = (ImageView)findViewById(R.id.myanimation);
final AnimationDrawable myAnimationDrawable
= (AnimationDrawable)myAnimation.getDrawable();
Button startAnimation = (Button)findViewById(R.id.startanimation);
Button stopAnimation = (Button)findViewById(R.id.stopanimation);
startAnimation.setOnClickListener(new Button.OnClickListener(){
@Override
public void onClick(View arg0) {
// TODO Auto-generated method stub
if(!myAnimationDrawable.isRunning()){
myAnimationDrawable.start();
}
}});
stopAnimation.setOnClickListener(new Button.OnClickListener(){
@Override
public void onClick(View arg0) {
// TODO Auto-generated method stub
if(myAnimationDrawable.isRunning()){
myAnimationDrawable.stop();
}
}});
}
}
main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical" >
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/hello" />
<Button
android:id="@+id/startanimation"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Start Animation" />
<Button
android:id="@+id/stopanimation"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Stop Animation" />
<ImageView
android:id="@+id/myanimation"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@anim/anim_android"
/>
</LinearLayout>

next:
- Set alpha of AnimationDrawable
- Detect AnimationDrawable.isRunning() to toggle start/stop animation
2 comments:
hi how would you achieve the same effect using one button
hello martin seal,
read HERE.
Post a Comment