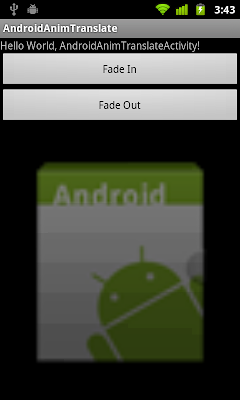
Create XML file for fade-in animation, /res/anim/fadein.xml.
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:interpolator="@android:anim/linear_interpolator">
<alpha
android:fromAlpha="0.1"
android:toAlpha="1.0"
android:duration="2000"
/>
</set>
Create XML file for fade-out animation, /res/anim/fadeout.xml.
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:interpolator="@android:anim/linear_interpolator">
<alpha
android:fromAlpha="1.0"
android:toAlpha="0.1"
android:duration="2000"
/>
</set>
Modify main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical" >
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/hello" />
<Button
android:id="@+id/fadein"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Fade In"/>
<Button
android:id="@+id/fadeout"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Fade Out"/>
<LinearLayout
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical"
android:gravity="center">
<ImageView
android:id="@+id/image"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:src="@drawable/ic_launcher" />
</LinearLayout>
</LinearLayout>
main activity
package com.exercise.AndroidAnimTranslate;
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
import android.view.animation.Animation;
import android.view.animation.AnimationUtils;
import android.widget.Button;
import android.widget.ImageView;
public class AndroidAnimTranslateActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
Button buttonFadeIn = (Button)findViewById(R.id.fadein);
Button buttonFadeOut = (Button)findViewById(R.id.fadeout);
final ImageView image = (ImageView)findViewById(R.id.image);
final Animation animationFadeIn = AnimationUtils.loadAnimation(this, R.anim.fadein);
buttonFadeIn.setOnClickListener(new Button.OnClickListener(){
@Override
public void onClick(View arg0) {
// TODO Auto-generated method stub
image.startAnimation(animationFadeIn);
}});
final Animation animationFadeOut = AnimationUtils.loadAnimation(this, R.anim.fadeout);
buttonFadeOut.setOnClickListener(new Button.OnClickListener(){
@Override
public void onClick(View arg0) {
// TODO Auto-generated method stub
image.startAnimation(animationFadeOut);
}});
}
}

Related articles:
- Animate falling action using Animation of translate
- Tween animation: rotate
- Animation of Scale
2 comments:
Thanks, very helpful.
Thank you, just what I needed :)
Post a Comment