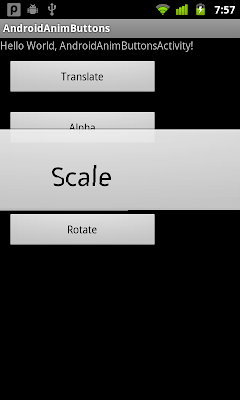
Create four XML files for animation:
/res/anim/anim_alpha.xml
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:interpolator="@android:anim/linear_interpolator">
<alpha
android:fromAlpha="1.0"
android:toAlpha="0.1"
android:duration="500"
android:repeatCount="1"
android:repeatMode="reverse" />
</set>
/res/anim/anim_rotate.xml
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:interpolator="@android:anim/linear_interpolator">
<rotate
android:fromDegrees="0"
android:toDegrees="360"
android:pivotX="50%"
android:pivotY="50%"
android:duration="500"
android:startOffset="0"
android:repeatCount="1"
android:repeatMode="reverse" />
</set>
/res/anim/anim_scale.xml
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:interpolator="@android:anim/linear_interpolator">
<scale
android:fromXScale="1.0"
android:toXScale="3.0"
android:fromYScale="1.0"
android:toYScale="3.0"
android:pivotX="50%"
android:pivotY="50%"
android:duration="500"
android:repeatCount="1"
android:repeatMode="reverse" />
</set>
/res/anim/anim_translate.xml
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:interpolator="@android:anim/linear_interpolator">
<translate
android:fromXDelta="0"
android:toXDelta="100%p"
android:duration="500"
android:repeatCount="1"
android:repeatMode="reverse"/>
</set>
main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical" >
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/hello" />
<Button
android:id="@+id/translate"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:layout_margin="10dp"
android:text="Translate" />
<Button
android:id="@+id/alpha"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:layout_margin="10dp"
android:text="Alpha" />
<Button
android:id="@+id/scale"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:layout_margin="10dp"
android:text="Scale" />
<Button
android:id="@+id/rotate"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:layout_margin="10dp"
android:text="Rotate" />
</LinearLayout>
Main activity:
package com.exercise.AndroidAnimButtons;
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
import android.view.animation.Animation;
import android.view.animation.AnimationUtils;
import android.widget.Button;
public class AndroidAnimButtonsActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
final Animation animTranslate = AnimationUtils.loadAnimation(this, R.anim.anim_translate);
final Animation animAlpha = AnimationUtils.loadAnimation(this, R.anim.anim_alpha);
final Animation animScale = AnimationUtils.loadAnimation(this, R.anim.anim_scale);
final Animation animRotate = AnimationUtils.loadAnimation(this, R.anim.anim_rotate);
Button btnTranslate = (Button)findViewById(R.id.translate);
Button btnAlpha = (Button)findViewById(R.id.alpha);
Button btnScale = (Button)findViewById(R.id.scale);
Button btnRotate = (Button)findViewById(R.id.rotate);
btnTranslate.setOnClickListener(new Button.OnClickListener(){
@Override
public void onClick(View arg0) {
arg0.startAnimation(animTranslate);
}});
btnAlpha.setOnClickListener(new Button.OnClickListener(){
@Override
public void onClick(View arg0) {
arg0.startAnimation(animAlpha);
}});
btnScale.setOnClickListener(new Button.OnClickListener(){
@Override
public void onClick(View arg0) {
arg0.startAnimation(animScale);
}});
btnRotate.setOnClickListener(new Button.OnClickListener(){
@Override
public void onClick(View arg0) {
arg0.startAnimation(animRotate);
}});
}
}

Next:
- Handle AnimationListener
Related:
- Start Animation in Activity start
More:
- Apply animation on buttons to start activity
19 comments:
This was a fantastic help. Thanks.
This helped me dude!! thanks for the post.
too much good example thanks
how can i make the animation run when the activity is started???
Custom Animation while switching Activity, using overridePendingTransition()
Thanks!:-)
You Are Ammmmmazing
thank you
BOoooo, just a litlte line to say how to 'declare' those four xml files should save hours of beginers... i will post as i find.
Really helpful, cheers
Very nice...
Thank you very much these tutorial helped me a lot. God bless you abundantly.
nice ...
how to retrieve and sava data in pdf format from database
Thanks for the share
Great post and still useful. Thank you
Thanks
For beautiful anim.
really amazing
Post a Comment